github.com/bgschiller/sync-and-swimA Tauri app to load music and audiobooks onto Shokz OpenSwim headphones. It offers three functions:
- Load music from a directory onto the headphones. Copying via Finder or Explorer tends to screw up the order of files (because the headphones sort by file timestamp instead of alphabetically). This tool copies files in the correct order.
- Slice long audio files, like podcasts or audiobooks, into smaller pieces. This makes it easier to skip forward or backward on a device with no screen and only three buttons.
- Find your place in a directory of audio files. Rather than starting from the beginning of the book if you lose your spot (like if the headphones accidentally turn on in your bag), this app uses binary search to find the last file you listened to.
github.com/bgschiller/vitest-matchers/tree/main/packages/unsettledCustom Vitest matcher for checking if a Promise is settled (either resolved or rejected).
If you expect a promise to be settled, you probably assert on its value: expect(p).resolves.toBe(value). But what if you want to assert that a promise is not settled? You can’t do that with the built-in matchers.
let resolve = null;
const p = new Promise((r) => {
resolve = r;
});
await expect(p).toBeUnsettled(); // passes
resolve(12);
await expect(p).not.toBeUnsettled(); // passes
github.com/bgschiller/cpp-subprocessA C++ library for safely (no exceptions!) invoking external commands and pipelines. 5x better than popen.h or your money back.
It’s not quite there yet, but the goal is to be able to write something like this:
auto res = (
Exec::shell("cat api/src/*.avdl") |
Exec::cmd("grep").arg("-oP").arg(R"(@since\((\d+)\))") |
Exec::cmd("tr").arg("-cd").arg("0-9\\n") |
Exec::cmd("sort").arg("-rn") |
Exec::cmd("head").arg("-n1")
).capture();
The killer app here is that you can avoid shell injection attacks because arguments are passed as separate strings, not as a single string that gets parsed by the shell. This is a common problem with system
and popen
in C++.
auto res = Redirection::Exec::cmd("jq").arg(jqFilter).stdin(body).capture();
This is all a shameless rip-off of Rust’s subprocess crate.
github.com/bgschiller/backsplashConversions between lat-lng, web mercator tiles, and pixel coordinates.
Useful to figure out which tiles you need to grab from a Tile Map Service depending on the lat-lng bounding box you’re interested in and the zoom level.
github.com/bgschiller/superdelegatePython port of Ruby ActiveSupport’s Model#delegate. Delegate methods and properties to child objects in a terse, explicit style.
github.com/bgschiller/alfred-clipboxAn Alfred workflow to save a screenshot to Trello and put a shareable link on your clipboard. Also supports screen recordings, text, and any file.
github.com/bgschiller/inspect.macroBabel plugin macro for loggin an expression and the result of that expression. Transforms
import inspect from "inspect.macro";
inspect(complicatedExpression(involving.many(parts * and * values)));
into
console.log(
"complicatedExpression(involving.many(parts * and * values)) →",
(function () {
try {
return complicatedExpression(involving.many(parts * and * values));
} catch (e) {
return "an error occurred: " + e;
}
})(),
);
github.com/bgschiller/vue-awaitRender blocks based on the status of a Promise. Demo at brianschiller.com/vue-await/.
<template>
<Await :p="prom">
<p>Waiting...</p>
<p slot="then" slot-scope="[result]">Success: {{ result }}</p>
<p slot="catch" slot-scope="[error]">Error: {{ error }}</p>
<p slot="none">(promise is null)</p>
</Await>
</template>
<script>
export default {
data() {
return {
prom: fetch("http://thecatapi.com/api/images/get"),
};
},
};
</script>
github.com/bgschiller/citrusA more convenient interface for doing Binary Linear Programming with PuLP. This package uses Python operator overloading to allow you to write
model.addConstraint(x & y == 1, "Both x and y must be true")
instead of
x_and_y = pulp.LpVariable("x_and_y", cat=pulp.Binary)
model.addConstraint(x_and_y == 1, "Both x and y must be true")
model.addConstraint(x_and_y <= x, "supporting constraint 1")
model.addConstraint(x_and_y <= y, "supporting constraint 2")
And similar for |
, negate
, implies
, maximum
and minimum
.
github.com/NREL/floorspace.jsFrontend for creating 2d building energy models. Integrated into NREL’s OpenStudio. Demo at nrel.github.io/floorspace.js/.
github.com/bgschiller/winnowA Python library to safely build powerful SQL queries from untrusted JSON structures. Demo code at bgschiller/winnow-demo, docs at winnow.readthedocs.io.
github.com/bgschiller/postgres_kernelInteract with your PostgreSQL db via a jupyter notebook.
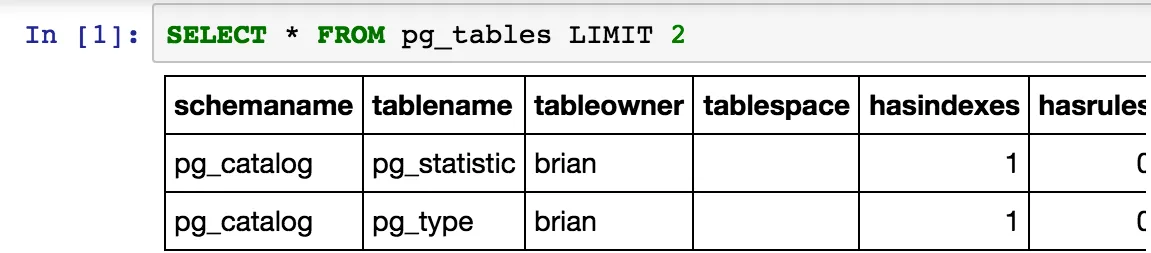
github.com/bgschiller/latex-thereforea LaTeX package to avoid the tedium of coming up with synonyms for ‘Therefore’ in your mathematical writing.
